Python for loop is probably the second most used control structure after the if-else statement. For loop in python runs over a fixed sequence and various operations are performed under that particular range. Python’s for loop is part of a definite iteration group. In this comprehensive tutorial, you will understand every component to build a for loop and how quickly and efficiently implement them in Python.
Table of Contents
- Why do we need for loop in Python
- Components of a for loop
- For loop in one line
- Iteration mode -For loop
- Else in for loop
- If-else inside a for loop
- Nested for loop in Python
- For loop on a list
- For loop on Strings
- For loop iterate through dictionary python
- Python for loop on set
- Python for loop on tuples
- Continue, break and pass
- Quiz – Python for loop
Why do we need for a loop in Python
Python for loop iterate over a fix sequence of data. A fixed sequence could be a list, or a string, or any other data structure in Python. A developer or a programmer can choose to go with a for loop, to iterate over the given sequence of data. It runs through (iterate over) each element in the sequence and performs some operation. In a short while, you will see all of them in action with the help to sample code.
Bottom line: When a fixed sequence is given, choose for loop for iteration.
Python for loop syntax
A for loop starts by typing “for” at the beginning followed by an iterator, then after a membership operator in and ends with the range (or collection). A colon (:) must be present at the end of the line, where “for loop” is declared, otherwise interpreter will throw an error.
A typical for loop syntax in Python is shown below,
for <iterator> in <range(x)>:
<body - execute during each iteration>
Components of a for loop
A for loop in python consists of an iterator, a specified range (a collection) and the body. Let’s understand each component in detail
Iterator in for loop
Iterator in the Python for loop helps to iterate from beginning till the end of the range. It starts from the first value of the range and iterates over each item until the last element.
for i in range(5):
body of for loop
In the above example, i is the iterator which starts from the 0th index (0) of range 5 until the last index (4).
Python Range () function
Range in Python creates a range object. An iterator uses this range object to loop over from beginning till the end. This range can also be one of the collections such as List, tuple, strings, sets and dictionary. You will see the usage of these collections in python for loop in the subsequent section.
Let’s understand what happens when you creates a range function in Python.
x = range(5)
print (x)
Output:
range(0,5)
print (list(x))
Output:
[0, 1, 2, 3, 4]
type (x)
Output:
<class ‘range’>
The default start value for the range function is 0, however, you can specify a start value.
The range (5) means a collection of 5 elements which are 0, 1, 2, 3, 4 (but not 5). When you pass the range object in len ( ) function, it will result in the number of elements, in this case, it will be 5.
print (len(x))
5
Let’s get a bit deeper into the range function in Python
Syntax: range (start value, end value, steps)
As you already know, the default start value is 0 and the default step (increment/decrement) value is 1. The “step” value can either be positive or negative. Detailed variations of the built-in range function are available at the official python documentation.
>>> list(range(10))
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> list(range(1, 11))
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
>>> list(range(0, 30, 5))
[0, 5, 10, 15, 20, 25]
>>> list(range(0, 10, 3))
[0, 3, 6, 9]
>>> list(range(0, -10, -1))
[0, -1, -2, -3, -4, -5, -6, -7, -8, -9]
>>> list(range(0))
[]
>>> list(range(1, 0))
[]
Body of Python for loop
The body of Python for loop contains the main execution part. When the iterator iterates over the range of elements, at every step the iterator holds a specific value. You can print each value of iterator in the following way,
for i in range(5):
print(i)
Output:
0
1
2
3
4
Now, you can implement your logic in the body section of for loop. Let’s say you want to add 5 to each element in the range (0,5). Python code for the same is,
for i in range(5):
print (i+5)
Output:
5
6
7
8
9
Flowchart – Python for loop
The following diagram shows Python for loop flow diagram.
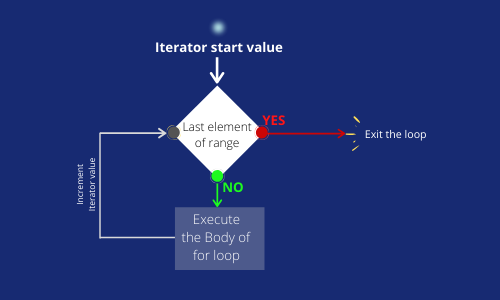
For loop in one line
Simple for loop in Python can also be written in one line. When the body of for loop is of just one line then the for loop can be written as shown below,
for i in range(5): print ("Current value of i is: " , i)
The normal version of the above code:
for i in range(5):
print ("Current value of i is: " , i)
Output:
The current value of i is: 0
The current value of i is: 1
The current value of i is: 2
The current value of i is: 3
The current value of i is: 4
Iteration mode
Python for loop can execute in two different ways,
- Membership mode [default mode]
- Index mode
Membership Mode – Python for loop
In membership mode, the iterator value is first confirmed in the range. Once the value is confirmed the body of the for loop gets executed.
for i in range(6):
print (i)
Foreach execution the current value of “i” is confirmed in the range object, Once the value is asserted, the body of for loop gets executed. Here “in” is a membership operator.
Index-based Iteration – Python for loop
In the Index mode of Iteration, the iterator acts as an index value.
x = [5, 8, 12, 56, 3]
for i in range(len(x)):
print (x[i])
Output:
5
8
12
56
3
Foreach iteration, i is asserted in the range(len(x)) and if the value is within the limit then the body section of for loop gets executed. Here x is a List and x[i] means the element of x at index i.
Else in for loop
Else statement is optional to place after Python for loop. Else is similar to that of the if-else statement, where else part executes those condition, which is not executed by the “if” part. You can use else to execute anything after the “for loop” is over.
Example
for i in range(5):
print(i)
else:
print("Out of for loop now")
If-else in for loop
Python for loop can also have if statement present in the body. The if statement used to perform some checks during each execution of the loop as shown in the example below,
for i in range(5):
if i == 3:
print ("Current value is 3")
else:
print ("This value is not 3")
Output:
This value is not 3
This value is not 3
This value is not 3
Current value is 3
This value is not 3
Nested for loop in Python
Nested loop means one loop inside another loop, same applies here. A nested Python for loop means one for loop inside another for-loop.
Let’s understand with an example,
for i in range(5):
for j in range(i+1):
print ('*',end=' ')
print ('\n')
Output:
*
* *
* * *
* * * *
* * * * *
In the above example, the outer loop (having i) start with 0. The inner loop executes until the range of (i+1) and prints the asterisk (*) with space next to it and before leaving the inner loop, it will also create a new line. The next execution starts from this new line and this keeps on happening until the range elements get exhausted.
For loop on a list
As you know about the List data structure, it is the collection of elements under one name tag. A Python for loop can iterate over each element of a list. An example of accessing list element using for loop is shown below,
list1 = [2,3,4,5]
for i in list1:
print("Current element is : {}".format(i))
Output:
Current element is : 2
Current element is : 3
Current element is : 4
Current element is : 5
For loop on Strings
String in python is also a collection of character (alphabet). Similar to the list, a for loop can iterate over the string (including blank space). Consider the string “Keep Learning and Keep Growing”. Now you can use a for loop to print each character individually as shown below,
s = "Keep Learning and Keep Growing"
for chr in s:
print (chr, end = ' , ')
Output:
K,e,e,p, ,L,e,a,r,n,i,n,g, ,a,n,d, ,K,e,e,p, ,G,r,o,w,i,n,g,
Another way of writing the same example is,
s = "Keep Learning and Keep Growing"
for i in range(len(s)):
print(s[i], end=' ')
Output:
K,e,e,p, ,L,e,a,r,n,i,n,g, ,a,n,d, ,K,e,e,p, ,G,r,o,w,i,n,g,
For loop iterate through dictionary python
I preassume that you are aware of the dictionary, in case you do not then check out the complete tutorial on the same. A for loop can also be used to iterate through the keys of the dictionary and will return you current key and the corresponding value. A typical example to use for loop over dictionary is shown below,
dict1 = {"Jan":1, "Feb":2, "Mar":3}
for val in dict1:p
print (val, dict1[val] )
Output:
Jan 1
Feb 2
Mar 3
For loop on set
I am also assuming that you know about set and in case you don’t then check out the complete tutorial on set. A for loop can be used to iterate over the set and will return you each element of the set, as shown in the example below,
set1 = {1, 2, 3, 4}
for val in set1:
print (val)
Output:
1
2
3
4
Python for loop on tuples
I am also assuming that you know about tuples and in case you don’t then check out the complete tutorial. A for loop can be used to iterate over the tuple and will return each element as shown in the example below,
tup1 = (1, 2, 3, 4)
for item in tup1:
print (item)
Output:
1
2
3
4
Continue, break and pass in for loop
You can consider these three terms namely continue, break and pass, as the controller of any loop. These are predefined keyword having a special instruction attached with it. Let’s understand each one of them in for loop context.
Continue
Continue keyword, when used in for loop, will return the control to the beginning of the loop. The “continue” will work when a certain condition is met during the execution and you want that specific condition to be ignored and keep the execution running.
my_string = "Welcome to aipython"
for chr in my_string:
if chr != " ":
print (chr, end='')
continue
Output:
Welcometoaipython
Break
A break is used to achieve an early exit from the for loop. A break can kick into the action as soon as a specific condition is met. The break condition will terminate the loop and do operations that are defined outside the loop.
my_list = ['Hi','hello','welcome','aipython','out','check']
for item in range(len(my_list)):
if my_list[item] != 'out':
print ("Loop is continuing")
else:
print ("Keyword OUT is found, Now exiting the loop")
break
Output:
Loop is continuing
Loop is continuing
Loop is continuing
Loop is continuing
Keyword OUT is found, Now exiting the loop
Pass
A pass keyword is very powerful as well as very helpful in Python. If you are designing a big application and you wish to define a specific section later, then you can use a pass. The pass keyword will skip the execution of that specific section. It can be applied in the loop as well as during user define function.
We will use the previous example but instead of terminating the program at “out” keyword, we would like to pass that block and keep executing till the last value of the list.
my_list = ['Hi','hello','welcome','aipython','out','check']
for item in range(len(my_list)):
if my_list[item] != 'out':
print ("Loop is continuing")
else:
print ("Keyword OUT is found, Now exiting the loop")
pass
Output
Loop is continuing
Loop is continuing
Loop is continuing
Loop is continuing
Keyword OUT is found, Now exiting the loop
Loop is continuing
Conclusion
Let recap it together.
After reading this comprehensive tutorial on Python for loop, you would have acquired the in-depth knowledge about for loop. You have learned to use for loop in your python code and use various features. To summarise it up, you have learned
- For loop components
- Iteration mode
- If-else in for loop
- Nested loops
- Loops on the various data structure in python
- Continue, break and pass statement
If you find this article worthy, then please like and also share it in your Network.
Keep Learning and Keep Growing !!
So much elaborative contents and that too Free of cost. sir i would say start python classes. I have also learned Python programming from udemy and they have not included as much details as you have included here.
Hats off… i will share this to my network.
keep it up