The ternary operator aims at reducing multiple lines of if-else complexity into a single line. It works upon, where the result of an expression (conditional expression) depends on the True or False value.
Bottom line: Consolidate (or condense) multi line expression in one line.
Table of Contents
Python If-else in one line
If-else can be re-written in one line using the ternary. Learn more about Python if-else statement.
Syntax-if-else
The syntax using Python if else one line
[execute_on_True] if [expression] else [execute_on_False]
The expression can consists of a comparison operator or logical operator. The final output of the expression should always be boolean (True or False)
The syntax without Ternary (in multiple lines)
if (expression):
execute_on_True
else:
execute_on_False
Tips to avoid mistake when using Ternary Operators in Python
- Don’t put a colon (:) at the end of the line, not required
- Remember the order of block
- Don’t put [ ] square bracket in actual code
- elif can not be used in the Ternary expression
- The complete statement can be assigned to a variable, as shown below
result = [execute_on_True] if [expression] else [execute_on_False]
Example and solution -if-else
Problem statement: Python program to print “Number is bigger” if the provided number (num1) is greater than 5, otherwise print “Number is smaller”.
Solution using Ternary
num1 = 6
print("Number is bigger") if num1 > 5 else print("Number is smaller")
output >> Number is bigger
Solution without Ternary
num1 = 6
if num1 > 5:
print("Number is bigger")
else:
print("Number is smaller")
Output -> Number is bigger
Ternary operator for Nested if-else
A nested if-else can also be easily handled by Ternary expression. To know more about Nested-if-else statement. A book on this topic is available online.
Syntax -Nested-if
The syntax for Nested if-else using ternary
[exp_1 True] if [exp_1] else [exp_2 True] if [exp_2] else [exp_2 False]
The syntax for Nested if-else without using Ternary
if (exp_1):
exp_1 True
else:
if (exp_2):
exp_2 True
else:
exp_2 False
Understand Nested if-else with the help of image given below.
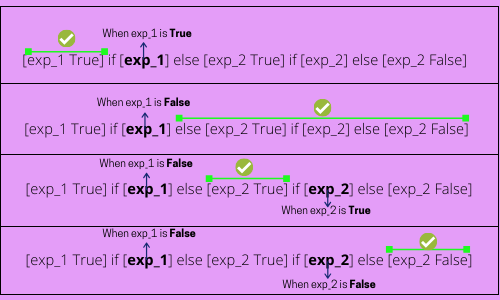
Example and solution -Nested-if
Problem statement: Python program to print “Number is bigger than 5” if the provided number (num1) is greater than 5, print “Number is less than 3” if the number is even less than 3, and finally, print “Number is bigger than 5” if both the condition is False.
Solution using Ternary
num1 = 4
print ("Number is bigger than 5") if num1 > 5 else print ("Number is less than 3") if num1 < 3 else print ("Number is bigger than 3")
output-> Number is bigger than 3
Solution without Ternary
num1 = 6
if (num1 > 5):
print ("Number is bigger than 5")
else:
if (num1 < 3):
print ("Number is less than 3")
else:
print ("Number is bigger than 3")
Output -> Number is bigger than 5
Variations of Ternary Operators
Ternary operator can be implemented with a few Python data structure such as Tuples and Dictionary.
Ternary expression using Tuples
Tuples can be used to create the ternary expression and the syntax for the same is shown below,
Syntax and example
(result_on_False, result_on_True) [ expression/condition ]
num1 = 10
print (("No", "Yes") [ num1 > 8 ])
Output-> Yes
Point to remember
- (“No”, “Yes”) is a tuple and should always be enclosed in bracket ( )
- The expression should always be written inside square bracket [ ]
A ternary operator using Dictionary
Dictionary can also be used to create the ternary expression and the syntax for the same is shown below,
Syntax and example
{True: Execute_on_true , False: Execute_on_false} [ expression/condition]
Let,s understand it,
Firstly, the expression gets executed and it will outcome a boolean value (either True or False). Secondly, the output boolean value is searched in the keys of the dictionary and once found the value of that key will be displayed as output.
{True: "Bigger" , False: "Smaller"} [ 10 < 21 ]
Output -> Bigger
Point to remember
- Since the dictionary is used in this expression so the keys (i.e, True & False) place can be interchanged and the result will not be affected.
- The expression should always be written inside square bracket [ ]
A ternary operator using Lambda function
Lambda function is one of the most beautiful things that is available within Python. Lambda function is more efficient (as it ensures the evaluation of one expression at a time) than using Tuples and Dictionary in ternary expression.
Syntax and example
(execute_lambda_on_False, execute_lambda_on_True ) [ expression ] ( )
a, b = 8, 3
( lambda : b-2, lambda : a+10 ) [ a > b ] ( )
Output -> 18
Point to remember
- Write both Lambda expression inside bracket ( )
- Write expression inside square bracket [ ]
- Don’t forget to put ( ) at the end of the expression
Keep Learning and Keep Growing !!
Hey! I just wanted to ask if you ever have any issues with hackers?
My last blog (wordpress) was hacked and I ended up losing several weeks of hard work due to
no backup. Do you have any methods to protect against hackers?